Android: How to use MockWebServer for Edge-cases Test, UI Test, and Unit Test together?

Testing on Android is troublesome especially when we need to cover some edge-cases like server returned unexpected data, certificate pining failure, and other network conditions.
Also, there are some common issues when we are conducting automated testing:
- The server is not stable, sometimes timeout
- Testing speed is slow because the server response is slow
- Some test cases alter the server states and cannot replay again
All these issues we can resolve together by using MockWebServer, as part of the OkHttp library provided by Square.
This article does not only show the basic usage of MockWebServer, but also shows you how we can easily mock the API response everywhere no matter when you run the application, run an instrumentation test, or run a unit test.
Basic Usage of MockWebServer
Add dependency
Add the dependency in your project by adding this to your build.gradle
file.
testImplementation 'com.squareup.okhttp3:mockwebserver:(insert latest version)'
Start the server
val mockServer = MockWebServer()
mockServer.start()
Mock response with enqueue()
Mock a response using enqueue(), it will enqueue one response each time:
val response = MockResponse().setResponseCode(200).setBody("{}")
mockServer.enqueue(mockedReponse)
After that, you just need to send the request to the MockWebServer URL, it will respond to you with the above mock response.
You can get the MockWebServer base URL below way, but as long as the HTTP scheme, host, and port match to MockWebServer, it will respond to you anyway.
val baseUrl = server.url(""); // http://localhost:33045
The host is fixed to localhost
. If you don’t calluseHttps()
, by default the HTTP scheme is http
also. The port is randomly given, if you need a fixed port, you can assign it when you start the server.
mockServer.start(8000)
Using enqueue()
is only convenient for unit tests because each time the API will be called one time. If you need the mock server to return you the same response every time the API is called. You need to useDispacher
.
Mock response with Dispatcher
The following is an example of using a Dispatcher
.
Use MockWebServer anywhere in the App
To be easy to explain how to archive it, I created a demo application and some demo videos by using the application.
Mock API response at run time:

Instrumentation Test with Mock API response:
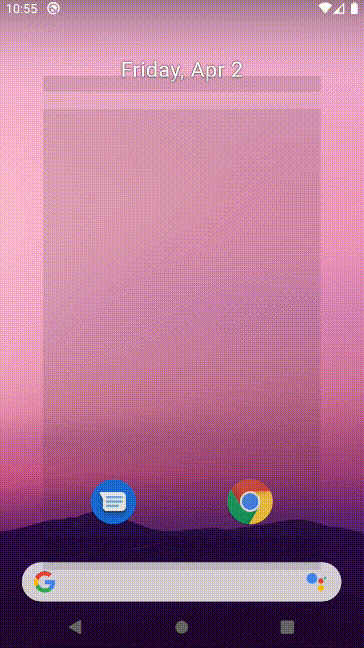
Let’s see how can we achieve it.
Add dependency
Firstly, we need to change the dependency from testImplementation
to debugImplemtation
, so that we can use MockWebServer inside the app.
implementation 'com.squareup.okhttp3:mockwebserver:(insert latest version)'
MockServerManager
To easily manage the MockWebServer, I created this manager to start and stop the mock server, enable or disable certain API mocking. When the API is enabled, a mock scenario is provided also to tell the mock server what kinds of data are returned.
Interceptor
How does OkHttp know which API needs to be mocked and which one doesn’t need? It is done by calling shouldMockApi()
from MockServerManager. If the API need to be mocked, the host will be changed to localhost
, and the port has to be the same port that MockWebServer used.
Dispatchers
Once the API call hits the localhost
and the same port with MockWebServer, the API call will route to MockWebServer instead of the real server. MockWebServer will ask the dispatcher for the MockResponse
in this case.
The following is an example to return different responses based on the scenario
input.
Start the mock server and enable API mocking
After that, you can start to mock any API as below at any place of your application, in an instrumentation test or unit test.